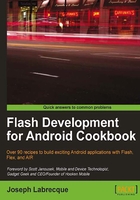
Using gestures to zoom a display object
Pinching and pulling are gestures that are often used on touch screens that support multitouch input. Bringing two fingers closer together will shrink an object, while spreading two fingers apart makes the object larger on the device.
How to do it...
This example draws a square within a Shape
object using the Graphics
API, adds it to the Stage
, and then sets up listeners for zoom gesture events in order to scale the Shape
appropriately:
- First, import the following classes into your project:
import flash.display.StageScaleMode; import flash.display.StageAlign; import flash.display.Stage; import flash.display.Sprite; import flash.display.Shape; import flash.events.TransformGestureEvent; import flash.ui.Multitouch; import flash.ui.MultitouchInputMode;
- Declare a
Shape
object, upon which we will perform the gestures:private var box:Shape;
- Next, construct a method to handle the creation of our
Sprite
and add it to theDisplayList:
protected function setupBox():void { box = new Shape(); box.graphics.beginFill(0xFFFFFF, 1); box.x = stage.stageWidth/2; box.y = stage.stageHeight/2; box.graphics.drawRect(-150,-150,300,300); box.graphics.endFill(); addChild(box); }
- Set the specific input mode for the multitouch APIs to support touch input by setting
Multitouch.inputMode
to theMultitouchInputMode.TOUCH_POINT
constant theMultitouchInputMode.TOUCH_POINT
constant and register anevent listener for theGESTURE_ZOOM
event. In this case, theonZoom
method will fire whenever the application detects a zoom gesture:protected function setupTouchEvents():void { Multitouch.inputMode = MultitouchInputMode.GESTURE; stage.addEventListener(TransformGestureEvent. GESTURE_ZOOM, onZoom); }
- To use the accepted behavior of pinch and zoom, we can adjust the scale of objects on stage based upon the scale factor returned by our event listener.
protected function onZoom(e:TransformGestureEvent):void { box.scaleX *= e.scaleX; box.scaleY *= e.scaleY; }
- The resulting gesture will affect our visual object in the following way:
Note
Illustrations provided by Gestureworks (www.gestureworks.com).
How it works...
As we are setting our Multitouch.inputMode
to gestures through MultitouchInputMode.GESTURE
, we are able to listen for and react to a host of predefined gestures. In this example, we are listening for the TransformGestureEvent.GESTURE_ZOOM
event in order to set the scale of our Shape
object. By multiplying the current scale properties by the scale values reported through our event, we can adjust the scale of our object based upon this gesture.
There's more...
Note here that we are drawing our square in such a way that the Shape
registration point is located in the center of the visible Shape
. It is important that we do this, as the DisplayObject
will scale up and down, based upon the registration point and transform point.
When using the drawing tools in Flash Professional, be sure to set the registration point of your MovieClip
symbol to be centered in order for this to work correctly.
See also...
TransformGestureEvent.GESTURE_ZOOM
is just one of a set of four primary transform gestures available to us when working with the Flash Platform runtimes and Android devices. Reference the following recipes for a complete overview of these gestures:
- Using gestures to pan a display object
- Using gestures to swipe a display object
- Using gestures to rotate a display object