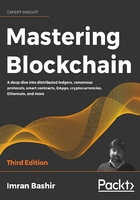
Blockchain
As we discussed in Chapter 1, Blockchain 101, a blockchain is a distributed ledger of transactions. Specifically, from Bitcoin's perspective, the blockchain can be defined as a public, distributed ledger holding a timestamped, ordered, and immutable record of all transactions on the Bitcoin network. Transactions are picked up by miners and bundled into blocks for mining. Each block is identified by a hash and is linked to its previous block by referencing the previous block's hash in its header.
The data structure of a Bitcoin block is shown in the following table:

The block header mentioned in the previous table is a data structure that contains several fields. This is shown in the following table:

The following diagram provides a detailed view of the blockchain structure. As shown in the following diagram, blockchain is a chain of blocks where each block is linked to its previous block by referencing the previous block header's hash. This linking makes sure that no transaction can be modified unless the block that records it and all blocks that follow it are also modified. The first block is not linked to any previous block and is known as the genesis block:

Figure 6.16: A visualization of blockchain, block, block header, transactions, and scripts
The preceding diagram shows a high-level overview of the Bitcoin blockchain. On the left-hand side, blocks are shown starting from bottom to top. Each block contains transactions and block headers, which are further magnified on the right-hand side. At the top, first, the block header is enlarged to show various elements within the block header. Then on the right-hand side, the Merkle root element of the block header is shown in magnified view, which shows how Merkle root is constructed.
We have discussed Merkle trees in detail previously. You can refer to Chapter 4, Public Key Cryptography, if you need to revise the concept.
Further down the diagram, transactions are also magnified to show the structure of a transaction and the elements that it contains. Also, note that transactions are then further elaborated to show what locking and unlocking scripts look like. The size (in bytes) of each field of block, header and transaction is also shown as a number under the name of the field.
Let's move on to discuss the first block in the Bitcoin blockchain: the genesis block.
The genesis block
This is the first block in the Bitcoin blockchain. The genesis block was hardcoded in the Bitcoin core software. In the genesis block, the coinbase transaction included a comment taken from The Times newspaper:
This message is a proof that the first Bitcoin block (genesis block) was not mined earlier than January 3rd, 2009. This is because the genesis block was created on January 3rd, 2009 and this news excerpt was taken from that day's newspaper.
The following representation of the genesis block code can be found in the chainparams.cpp
file available at https://github.com/Bitcoin/Bitcoin/blob/master/src/chainparams.cpp:
static CBlock CreateGenesisBlock(uint32_t nTime, uint32_t nNonce, uint32_t nBits, int32_t nVersion, const CAmount& genesisReward)
{
const char* pszTimestamp = "The Times 03/Jan/2009 Chancellor on brink of second bailout for banks";
const CScript genesisOutputScript = CScript() << ParseHex("04678afdb0fe5548271967f1a67130b7105cd6a828e03909a67962e0ea1f61deb649f6bc3f4cef38c4f35504e51ec112de5c384df7ba0b8d578a4c702b6bf11d5f") << OP_CHECKSIG;
return CreateGenesisBlock(pszTimestamp, genesisOutputScript, nTime, nNonce, nBits, nVersion, genesisReward);
}
Bitcoin provides protection against double-spending by enforcing strict rules on transaction verification and via mining. Transactions and blocks are added to the blockchain only after the strict rule-checking explained in the Transaction validation section on successful PoW solutions.
Block height is the number of blocks before a particular block in the blockchain. PoW is used to secure the blockchain. Each block contains one or more transactions, out of which the first transaction is the coinbase transaction. There is a special condition for coinbase transactions that prevent them from being spent until at least 100 blocks have passed in order to avoid a situation where the block may be declared stale later on.
Stale and orphan blocks
Stale blocks are old blocks that have already been mined. Miners who keep working on these blocks due to a fork, where the longest chain (main chain) has already progressed beyond those blocks, are said to be working on a stale block. In other words, these blocks exist on a shorter chain, and will not provide any reward to their miners.
Orphan blocks are a slightly different concept. Their parent blocks are unknown. As their parents are unknown, they cannot be validated. This problem occurs when two or more miners discover a block at almost the same time. These are valid blocks and were correctly discovered at some point in the past but now they are no longer part of the main chain. The reason why this occurs is that if there are two blocks discovered at almost the same time, the one with a larger amount of PoW will be accepted and the one with the lower amount of work will be rejected. Similar to stale blocks, they do not provide any reward to their miners.
We can see this concept visually in the following diagram:

Figure 6.17: Orphan and stale blocks
In the preceding introduction to stale blocks, we introduced a new term, a fork. A fork is a condition that occurs when two different versions of the blockchain exist. It is acceptable in some conditions, and detrimental in a few others. There are different types of forks that can occur in a blockchain:
- Temporary forks
- Soft forks
- Hard forks
Because of the distributed nature of Bitcoin, network forks can occur inherently. In cases where two nodes simultaneously announce a valid block, it can result in a situation where there are two blockchains with different transactions. This is an undesirable situation but can be addressed by the Bitcoin network only by accepting the longest chain. In this case, the smaller chain will be considered orphaned. If an adversary manages to gain control of 51% of the network hash rate (computational power), then they can impose their own version of the transaction history.
Forks in a blockchain can also occur with the introduction of changes to the Bitcoin protocol. In the case of a soft fork, a client that chooses not to upgrade to the latest version supporting the updated protocol will still be able to work and operate normally. In this case, new and previous blocks are both acceptable, thus making a soft fork backward compatible. Miners are only required to upgrade to the new soft fork client software in order to make use of the new protocol rules. Planned upgrades do not necessarily create forks because all users should have updated already.
A hard fork, on the other hand, invalidates previously valid blocks and requires all users to upgrade. New transaction types are sometimes added as a soft fork, and any changes such as block structure changes or major protocol changes result in a hard fork.
As Bitcoin evolves and new upgrades and innovations are introduced in it, the version associated with blocks also changes. These versions introduce various security parameters and new features. The latest Bitcoin block version is 4, which was proposed with BIP65 and has been used since Bitcoin Core client 0.11.2. Since the implementation of BIP9, bits in the nVersion field are used to indicate soft-fork changes.
Details of BIP0065 are available at https://github.com/Bitcoin/bips/blob/master/bip-0065.mediawiki.
Size of the blockchain
Bitcoin is an ever-growing chain of blocks and is increasing in size. The current size of the Bitcoin blockchain stands at approximately 269 GB. The following figure shows the increase in size of the blockchain over time:

Figure 6.18: Size of Bitcoin blockchain over time
As the chain grows and more miners are added to the network, the network difficulty also increases.
Network difficulty
Network difficulty refers to a measure of how difficult it is to find a new block, or in other words, how difficult it is to find a hash below the given target.
New blocks are added to the blockchain approximately every 10 minutes, and the network difficulty is adjusted dynamically every 2,016 blocks in order to maintain a steady addition of new blocks to the network.
Network difficulty is calculated using the following equation:
Target = Previous target * Time/2016 * 10 minutes
Difficulty and target are interchangeable and represent the same thing. The Previous target represents the old target value, and Time is the time spent to generate the previous 2,016 blocks. Network difficulty essentially means how hard it is for miners to find a new block; that is, how difficult the hashing puzzle is now.
In the next section, mining is discussed, which will explain how the hashing puzzle is solved.