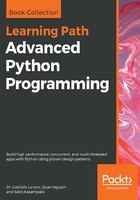
Mathematical operations
NumPy includes the most common mathematical operations available for broadcasting, by default, ranging from simple algebra to trigonometry, rounding, and logic. For instance, to take the square root of every element in the array, we can use numpy.sqrt, as shown in the following code:
np.sqrt(np.array([4, 9, 16]))
# Result:
# array([2., 3., 4.])
The comparison operators are useful when trying to filter certain elements based on a condition. Imagine that we have an array of random numbers from 0 to 1, and we want to extract all the numbers greater than 0.5. We can use the > operator on the array to obtain a bool array, as follows:
a = np.random.rand(5, 3)
a > 0.3
# Result:
# array([[ True, False, True],
# [ True, True, True],
# [False, True, True],
# [ True, True, False],
# [ True, True, False]], dtype=bool)
The resulting bool array can then be reused as an index to retrieve the elements greater than 0.5:
a[a > 0.5]
print(a[a>0.5])
# Output:
# [ 0.9755 0.5977 0.8287 0.6214 0.5669 0.9553 0.5894
0.7196 0.9200 0.5781 0.8281 ]
NumPy also implements methods such as ndarray.sum, which takes the sum of all the elements on an axis. If we have an array of shape (5, 3), we can use the ndarray.sum method to sum the elements on the first axis, the second axis, or over all the elements of the array, as illustrated in the following snippet:
a = np.random.rand(5, 3)
a.sum(axis=0)
# Result:
# array([ 2.7454, 2.5517, 2.0303])
a.sum(axis=1)
# Result:
# array([ 1.7498, 1.2491, 1.8151, 1.9320, 0.5814])
a.sum() # With no argument operates on flattened array
# Result:
# 7.3275
Note that by summing the elements over an axis, we eliminate that axis. From the preceding example, the sum on the axis 0 produces an array of shape (3,), while the sum on the axis 1 produces an array of shape (5,).