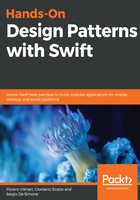
Using unowned
In our particular example, we cannot guarantee the duration of the life cycle of our objects, so unowned is unfit us example. Also, unowned provides fewer guarantees than weak, in terms of safety, can't be applied to Optionals. If you can't use weak for logic reasons, then there's little chance you'll be able to use unowned.
Let's investigate another piece of code involving credit cards, borrowed from Chapter 1, Refreshing the Basics.
First, let's see the code without the unowned modifier under the memory debugger:
class Card {
let owner: Person
init(_ owner: Person) {
self.owner = owner
}
}
class Person {
let name: String
var cards = [Card]()
init(name: String) {
self.name = name
}
}
func runTests() {
let batman = Person(name: "Batman")
batman.cards.append(Card(batman))
batman.cards.append(Card(batman))
batman.cards.append(Card(batman))
}
runTests()
This simple program should not leave any objects behind after runTests() finishes running; however, because we have a strong reference cycle between Person and Card, that is not the case, and all of the created objects will leak:

All of our objects have leaked, as shown in the preceding screenshot. This example is perfect for using unowned. The Card object can't live without an owner. So, whenever the owner is deallocated, all of the cards should be destroyed as well, as we'll never reference a card without its owner. We need to update our Card class to reflect that the Card objects are not retaining their owner:
class Card {
unowned let owner: Person
init(_ owner: Person) {
self.owner = owner
}
}
With this addition, the card cannot be allocated without a Person, and this person has to exist to be a valid card. In the next section, you'll see the issues that unowned can cause, and how we can prevent them.