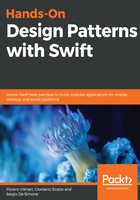
Thread safety through serial queues
Sometimes, in your code, you want to guarantee that no two concurrent threads will write to the same memory. You can use the @synchronized(self) section in Objective-C, but in Swift, you can leverage Dispatch and serial queues. As you saw in the previous section, the execution order will be preserved, as well as additional guarantees that the previous task enqueued on a serial queue will be completed before the next one starts.
You can ensure that access to the balance property of CreditCard is thread-safe, which guarantees that no other thread or code is writing while you are reading the value:
class CreditCard {
private let queue = DispatchQueue(label: "synchronization.queue")
private var _balance: Int = 0
var balance: Int {
get {
return queue.sync { _balance }
}
set {
queue.sync { _balance = newValue }
}
}
}
In the preceding example, we use a shadow variable (balance) to provide public access to the underlying _balance. The shadow variable will guarantee that the _balance object/variable will always be accessed in a thread safe manner.