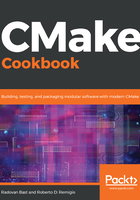
上QQ阅读APP看书,第一时间看更新
How to do it
These are the detailed steps to follow in CMakeLists.txt:
- As in Recipe 8, Controlling compiler flags, we specify the minimum required version of CMake, project name, and language, and declare the geometry library target:
cmake_minimum_required(VERSION 3.5 FATAL_ERROR)
project(recipe-10 LANGUAGES CXX)
add_library(geometry
STATIC
geometry_circle.cpp
geometry_circle.hpp
geometry_polygon.cpp
geometry_polygon.hpp
geometry_rhombus.cpp
geometry_rhombus.hpp
geometry_square.cpp
geometry_square.hpp
)
- We decide to compile the library with the -O3 compiler optimization level. This is set as a PRIVATE compiler option on the target:
target_compile_options(geometry
PRIVATE
-O3
)
- Then, we generate a list of source files to be compiled with lower optimization:
list(
APPEND sources_with_lower_optimization
geometry_circle.cpp
geometry_rhombus.cpp
)
- We loop over these source files to tune their optimization level down to -O2. This is done using their source file properties:
message(STATUS "Setting source properties using IN LISTS syntax:")
foreach(_source IN LISTS sources_with_lower_optimization)
set_source_files_properties(${_source} PROPERTIES COMPILE_FLAGS -O2)
message(STATUS "Appending -O2 flag for ${_source}")
endforeach()
- To make sure source properties were set, we loop once again and print the COMPILE_FLAGS property on each of the sources:
message(STATUS "Querying sources properties using plain syntax:")
foreach(_source ${sources_with_lower_optimization})
get_source_file_property(_flags ${_source} COMPILE_FLAGS)
message(STATUS "Source ${_source} has the following extra COMPILE_FLAGS: ${_flags}")
endforeach()
- Finally, we add the compute-areas executable target and link it against the geometry library:
add_executable(compute-areas compute-areas.cpp)
target_link_libraries(compute-areas geometry)
- Let us verify that the flags were correctly set at the configure step:
$ mkdir -p build
$ cd build
$ cmake ..
...
-- Setting source properties using IN LISTS syntax:
-- Appending -O2 flag for geometry_circle.cpp
-- Appending -O2 flag for geometry_rhombus.cpp
-- Querying sources properties using plain syntax:
-- Source geometry_circle.cpp has the following extra COMPILE_FLAGS: -O2
-- Source geometry_rhombus.cpp has the following extra COMPILE_FLAGS: -O2
- Finally, also check the build step with VERBOSE=1. You will see that the -O2 flag gets appended to the -O3 flag but the last optimization level flag (in this case -O2) "wins":
$ cmake --build . -- VERBOSE=1