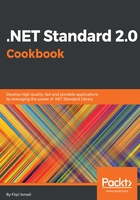
How it works...
Now we have a working WPF application that uses a .NET Standard 2.0 library as its source. Let's have a look at the steps we followed. In steps 1 to 7, we opened the previously built .NET Standard 2.0 library project and the solution. After that, we added a Windows Presentation Foundation project to the solution. As you know, WPF is a UI framework that runs on top of the .NET Framework.
From steps 9 to 13, we just created the user interface for our application. Then, in step 12, we changed some default properties of the controls we added. Giving meaningful names is a very good practice as it helps you to build readable code. In step 20, we just double-clicked on a control, in this case the button, to open the code windows. By default, Visual Studio chooses an event for us. Mainly, it chooses a commonly used event and, in this case, it's the click event of the button.
In step 22, we referenced the .NET Standard 2.0 library to the code. This will allow you to access its available methods in the WPF application. In step 23, we have the actual running code for the button click event. In the first line, we created an instance of LittleShop() and stored it in a variable. Then we used the GetFruitsList() method to get the list of fruits and stored it in a variable. Then we looped through all the available items in the fruits variable.
foreach (var fruit in fruits)
{
FruitsList.Items.Add(fruit);
}
In the previous code for the fruits variable, there is a List collection. foreach will loop through each item inside the List collection stored in the fruits variable. And inside the loop, a FruitsList list box control adds each item in the fruits collection, which is stored in the fruit variable.
FruitsList.Items.Add("--------");
FruitsList.Items.Add($"Item Count: {fruits.Count}");
FruitsList.Items.Add($"Capacity: {fruits.Capacity}");
After we have added each item to the list box (FruitsList), we have added a string that displays the number of items in the List collection. We have used the Count property in the List collection to get that information. And, in the last line of code, we picked the Capacity of the List collection. The capacity property gets or sets the total number of elements the internal data structure can hold without resizing.
In step 26, we created an instance of the LittleShop() class and used the GetShopItems() method to get the items returned as an ArrayList(). Then we used a for loop to get the items inside the second list box. The rest is the same as we did with the List collection.