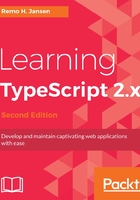
Variables, basic types, and operators
The basic types are boolean, number, string, array, tuple, Object, object, null, undefined, {}, void, and enumerations. Let's learn about each of these basic types:
Data type |
Description |
Boolean |
Whereas the string and number data types can have a virtually unlimited number of different values, the boolean data type can only have two. They are the literals: true and false. A boolean value is a truth value; it specifies whether the condition is true or not: let isDone: boolean = false; |
Number |
As in JavaScript, all numbers in TypeScript are floating-point values. These floating-point numbers get the type number: let height: number = 6; |
String |
We use the string data type to represent text in TypeScript. You include string literals in your scripts by enclosing them in single or double quotation marks. Double quotation marks can be contained in strings surrounded by single quotation marks and single quotation marks can be contained in strings surrounded by double quotation marks: let name: string = "bob"; name = 'Smith'; |
Array |
We use the array data type to represent a collection of values. The array type can be written using two different syntax styles. We can use the type of the elements in the array followed by brackets [] to annotate a collection of that element type: let list: number[] = [1, 2, 3]; The second syntax style uses a generic array type named Array<T>: let list: Array<number> = [1, 2, 3]; |
Tuple |
Tuple types can be used to represent an array with a fixed number of elements with different types where the type is known. For example, we can represent a value as a pair of a string and a number: let x: [string, number]; x = ["hello", 10]; // OK x = ["world", 20]; // OK x = [10, "hello"]; // Error x = [20, "world"]; // Error |
Enum |
We use enumerations to add more meaning to a set of values. Enumerations can be numeric or text-based. By default, numeric enumerations assign the value 0 to the first member in the enumeration and increase it by one for each of the members in the enumeration: enum Color {Red, Green, Blue}; let c: Color = Color.Green; |
Any |
All types in TypeScript are subtypes of a single top type called the any type. The any keyword references this type. The any type eliminates most of the TypeScript type checks and represents all the possible types: let notSure: any = 4; // OK notSure = "maybe a string instead"; // OK notSure = false; // OK The any type can be useful while migrating existing JavaScript code to TypeScript, or when we know some details about a type but we don't know all its details. For example, when we know that a type is an array, but we don't know the type of the elements in such an array: let list: any[] = [1, true, "free"]; list[1] = 100; |
object (lowercase) |
The object type represents any non-primitive type. The following types are considered to be primitive types in JavaScript: boolean, number, string, symbol, null, and undefined. |
Object (uppercase) |
In JavaScript, all objects are derived from the Object class. Object (uppercase) describes functionality that is common to all JavaScript objects. That includes the toString() and the hasOwnProperty() methods, for example. |
Empty object type {} |
This describes an object that has no members of its own. TypeScript issues a compile-time error when you try to access arbitrary properties of such an object: const obj = {}; obj.prop = "value"; // Error |
Null and undefined |
In TypeScript, both undefined and null are types. By default, null and undefined are subtypes of all other types. That means you can assign null and undefined to something like a number. However, when using the --strictNullChecks flag, null and undefined are only assignable to void and their respective types. |
Never |
The never type is used in the following two places:
function impossibleTypeGuard(value: any) { if ( typeof value === "string" && typeof value === "number" ) { value; // Type never } } |
Void |
In some ways the opposite of any is void, the absence of having any type at all. You will see this as the return type of functions that do not return a value: function warnUser(): void { console.log("This is my warning message"); } |
In TypeScript and JavaScript, undefined is a property in the global scope that is assigned as a value to variables that have been declared but have not yet been initialized. The value null is a literal (not a property of the global object) and it can be assigned to a variable as a representation of no value:
let testVar; // variable is declared but not initialized consoe.log(testVar); // shows undefined console.log(typeof testVar); // shows undefined let testVar = null; // variable is declared, and null is assigned as its value cosole.log(testVar); // shows null console.log(typeof testVar); // shows object