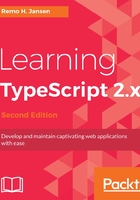
Optional static type annotations
TypeScript allows us to use type annotations to overcome the scenarios in which the type inference system is not powerful enough to automatically detect the type of a variable.
Let's consider the add function one more time:
function add(a, b) {
return a + b;
}
The type of the function add is inferred as the following type:
(a: any, b: any) => any;
The preceding type is a problem because the usage of the any type effectively prevents the TypeScript compiler from detecting certain errors. For example, we might expect the add function to add two numbers:
let result1 = add(2, 3); // 5
However, if we pass a string as input, we will encounter an unexpected result:
let result2 = add("2", 3); // "23"
The preceding error can happen very easily if, for example, the arguments provided to the add function have been extracted from an HTML input and we forget to parse them as a number.
We can fix the add function by adding optional type annotations:
function add(a: number, b: number): number {
return a + b;
}
We can add an optional type annotation by adding a colon (:) after the declaration of a variable followed by the type:
let myVariable: string = "Hello";
Now that the type of the arguments of the add function are number, instead of any, the TypeScript compiler will be able to detect potential issues if we provide arguments of the worn type:
let result1 = add(2, 3); // OK
let result2 = add("2", 3); // Error