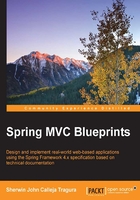
The project development
All the nuts and bolts involved in developing the PWP from scratch will be discussed in this topic. Each of the four web pages, including their internal processes, will be scrutinized using the codes of the project. The Spring MVC concepts will focus on the following areas:
- Configuration of
DispatcherServlet
- Configuration of Spring container
- Creating controllers
- Types of attributes
- Validation
- Type conversion and transformation
- E-mail support configuration
- Views and
ViewResolvers
Configuring the DispatcherServlet
We start creating the Spring MVC project by configuring the DispatcherServlet API class. The Spring MVC framework has the DispatcherServlet
at the center of all request and response transactions as illustrated in the preceding figure.
From the point of view of the PWP, the DispatcherServlet
starts receiving requests when the user starts running pages on the web browser. The processes are enumerated as follows:
- When the container receives a request from a path, the
DispatcherServlet
checks whose controller is mapped to the path name. - Then, the controller acknowledges the request with the appropriate service methods (for example,
GET
,POST
,PUT
,HEAD
), executes the appropriate transaction method with the given model(s), and then returns the view name to theDispatcherServlet
. - Then, the
DispatcherServlet
checks which type of view resolver has been configured from its container. Through the view resolver, theDispatcherServlet
will know the appropriate view that matches the given request. - Finally, the
DispatcherServlet
will process the transport of model data to the view for presentation or rendition.
The PWP has the following configuration for the DispatcherServlet
:
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>ChapterOne</display-name> <!-- Declare Spring DispatcherServlet --> <servlet> <servlet-name>pwp</servlet-name> <servlet-class> org.springframework.web.servlet.DispatcherServlet </servlet-class> </servlet> <servlet-mapping> <servlet-name>pwp</servlet-name> <url-pattern>*.html</url-pattern> </servlet-mapping> <!-- Spring accepted extension declared here below --> <mime-mapping> <extension>png</extension> <mime-type>image/png</mime-type> </mime-mapping> </web-app>
Just like any typical JEE servlet, the tags <servlet>
and <servlet-mapping>
are used to declare the dispatcher servlet DispatcherServlet
:
<servlet> <servlet-name>pwp</servlet-name> <servlet-class> org.springframework.web.servlet.DispatcherServlet </servlet-class> </servlet> <servlet-mapping> <servlet-name>pwp</servlet-name> <url-pattern>*.html</url-pattern> </servlet-mapping>
The <servlet-name>
tag does not only stand for the name of the servlet, but is also related to the name of a Spring container which will be tackled later. The <url-pattern>
indicates which type of valid URLs will be recognized by the DispatcherServlet
during request-response transactions. In our preceding configuration, it shows that all URL must have an extension .html
in order for the requests to be processed by the servlet.
When it comes to file types, the DispatcherServlet
only considers content types declared with the <mime-mapping>
tag. In this project, we only have PNG files needed by the portal.
<mime-mapping> <extension>png</extension> <mime-type>image/png</mime-type> </mime-mapping>