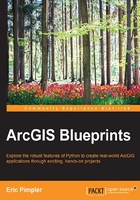
上QQ阅读APP看书,第一时间看更新
Coding the VisualizeMigration tool
In this final section, you'll create a new tool in the Migration Patterns toolbox that can be used to visualize elk migration patterns in one-week increments that have beginning and end dates, as specified through the tool. The tool will also export maps in the PDF format for each week:
- Import the
arcpy.mapping
anddatetime
modules that will be used in this class:import arcpy import arcpy.mapping as mapping import csv import os import datetime
- Create a new tool called
VisualizeMigration
by copying and pasting the existingImportCollarData
code and then renaming the class toVisualizeMigration
. - In the
VisualizeMIgration
class, set thelabel
anddescription
properties, as shown in the following code through the__init__
method:class VisualizeMigration(object): def __init__(self): """Define the tool (tool name is the name of the class).""" self.label = "Visualize Elk Migration" self.description = "Visualize Elk Migration" self.canRunInBackground = False
- You'll need two parameters to capture the
start
andend
dates. Add theParameter
objects, as shown in the following code through the getParameterInfo() method:def getParameterInfo(self): param0 = arcpy.Parameter(displayName = "Begin Date", \ name="beginDate", \ datatype="GPDate", \ parameterType="Required",\ direction="Input") param1 = arcpy.Parameter(displayName = "End Date", \ name="endDate", \ datatype="GPDate",\ parameterType="Required",\ direction="Input") params = [param0, param1] return params
- Capture the
start
andend
data parameter values in theexecute()
method:def execute(self, parameters, messages): """The source code of the tool.""" beginDate = parameters[0].valueAsText endDate = parameters[1].valueAsText
- Split the
day
,month
, andyear
values for thestart
andend
dates:def execute(self, parameters, messages): """The source code of the tool.""" beginDate = parameters[0].valueAsText endDate = parameters[1].valueAsText #begin date lstBeginDate = beginDate.split("/") beginMonth = int(lstBeginDate[0]) beginDay = int(lstBeginDate[1]) beginYear = int(lstBeginDate[2]) #end date lstEndDate = endDate.split("/") endMonth = int(lstEndDate[0]) endDay = int(lstEndDate[1]) endYear = int(lstEndDate[2])
- Get the current
MapDocument
,DataFrame
, andDataFrameTime
objects:def execute(self, parameters, messages): """The source code of the tool.""" beginDate = parameters[0].valueAsText endDate = parameters[1].valueAsText #begin date lstBeginDate = beginDate.split("/") beginMonth = int(lstBeginDate[0]) beginDay = int(lstBeginDate[1]) beginYear = int(lstBeginDate[2]) #end date lstEndDate = endDate.split("/") endMonth = int(lstEndDate[0]) endDay = int(lstEndDate[1]) endYear = int(lstEndDate[2]) mxd = mapping.MapDocument("current") df = mapping.ListDataFrames(mxd, "Layers")[0] dft = df.time
- Set the
currentTime
andendTime
properties on theDataFrameTime
object. This will set the boundaries of the visualization and map export:mxd = mapping.MapDocument("current") df = mapping.ListDataFrames(mxd, "Layers")[0] dft = df.time dft.currentTime = datetime.datetime(beginYear, beginMonth, beginDay) dft.endTime = datetime.datetime(endYear, endMonth, endDay)
- In the last section of this method, you will create a loop that accomplishes several tasks. The loop will operate between the
start
andend
dates, set the visible features to the current date, dynamically set the title to the current date, export a PDF file, and reset thecurrentTime
property to the next day. Add theWHILE
loop just below the last line of code you wrote in the last step. Note that you will have to hardcode a path to the output folder where the PDF files will be created. If you are so inclined, you may want to convert this into a parameter that is provided as input from the user:while dft.currentTime <= dft.endTime: for el in mapping.ListLayoutElements(mxd, "TEXT_ELEMENT", "*title*"): el.text = "Elk Migration Pattern: " + str(dft.currentTime).split()[0] fileName = str(dft.currentTime).split(" ")[0] + ".pdf" mapping.ExportToPDF(mxd,os.path.join(r"c:\ArcGIS_Blueprint_Python\ch2", fileName)) arcpy.AddMessage("Exported " + fileName) dft.currentTime = dft.currentTime + dft.timeStepInterval
- Check your code against the solution file found at
C:\ArcGIS_Blueprint_Python\solutions\ch2\VisualizeMigration.py
to make sure you have coded everything correctly. - Close your code editor.
- In ArcMap, open
C:\ArcGIS_Blueprint_Python\ch2\ElkMigration.mxd
. Open the Catalog view and execute the Visualize Elk Migration tool. You will be prompted to enter thestart
andend
dates, as shown in the following screenshot. The data for this particular elk spans the period between January 17, 2005 to November 4, 2005. To keep it simple, enter a fairly small time period, such as1/18/2005
to2/18/2005
. - Click on OK to execute the tool. If everything has been coded correctly, the progress dialog should be updated as each day is visualized and exported to a PDF file.
- After the execution, you can check the
C:\ArcGIS_Blueprint_Python\ch2
folder to see the output files. Each file should appear similar to what is shown in the following screenshot: