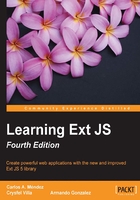
Working with the store
As mentioned before, a store is a collection of models that acts as a client cache to manage our data locally. We can use this collection to perform tasks such as sorting, grouping, and filtering the models in a very easy way. We can also pull data from our server using one of the available proxies and a reader to interpret the server response and fill the collection.
A store is usually added to widgets/components to display data. Components such as the grid, tree, combo box, or data view use a store to manage the data. We will learn about these components in future chapters. If we create a custom widget, we should use a store to manage the data too. This is why this chapter is really important; we use models and stores to deal with the data.
In order to create a store, we need to use the Ext.data.Store
class. The following example will use the Customer
model that we already have worked, and will extend the store to create a collection of customers:
Ext.define('MyApp.store.Customers',{ extend : 'Ext.data.Store', //Step 1 model : 'Myapp.model.Customer' //Step 2 });
The steps are explained as follows:
- Step 1: To define a store, we need to extend from the
Ext.data.Store
class. This class is responsible for dealing with the models. - Step 2: We associated the model that our store will be using. It is required to specify a valid
model
class; in this case, we're using ourCustomer
class that we have been working on in the previous example.
Once we have our store class defined, we are going to create an HTML page to run our test. Let's import the Ext
library, our Customer
model, and our Customers
store:
var store = Ext.create("MyApp.store.Customers"); //counting the elements in the store console.log(store.count());
We can use the create
method to instantiate our store
class; in this example, we don't need to pass any parameter, but we could do it as any other class.
If we would like to know the number of items that are contained in our store, we can use the count
method. In this case, we're printing the number returned on the JavaScript console, which is zero, because our store is empty at this moment.
Adding new elements
Adding elements to the collection is very simple. We need to create a Customer
model with data, and we will use the add
or insert
method to add the new item to our store, as shown in the following code:
//Step 1 (define /create new model instance) var mynewcustomer = Ext.create('Myapp.model.Customer',{ id: 10001, name: 'Acme corp', phone: '+52-01-55-4444-3210', website : 'www.acmecorp.com', status: 'Active', clientSince: '2010-01-01 14:35', contractInfo:{ id:444, contractId:'ct-001-444', documentType:'PDF' } }); store.add(mynewcustomer); //Step 2 console.log("Records in store:" + store.getCount() );
The steps are explained as follows:
- Step 1: We created the model that we want to add to our store; we also set values to some of the fields.
- Step 2: We executed the
add
method to append our model to the collection. It's important to know that using theadd
method will always insert the model in the last position of the collection.
Finally, we count our items again and we will see a number 1 in our JavaScript console.
We can also add a new item by just sending an object containing the data, and the add
method will create the model
instance for us, as shown in the following example:
//Method 2 for add Records store.add({ id: 10002, name: 'Candy Store LTD', phone: '+52-01-66-3333-3895', website : 'www.candyworld.com', status: 'Active', clientSince: '2011-01-01 14:35', contractInfo:{ id:9998, contractId:'ct-001-9998', documentType:'DOCX' } }); console.log("Records in store:" + store.getCount());
When running the previous code, we will see a number 2 in the JavaScript console.
We can even add many items at once by passing an array of models to the add
method, as shown in the following example:
// Method 3 for add multiple records var mynewcustomer = Ext.create('Myapp.model.Customer', { ...}); var mynewcustomerb = Ext.create('Myapp.model.Customer', { ...}); store.add([mynewcustomer, mynewcustomerb]); console.log("Records in store:" + store.getCount());
We have added two models in the same method call, but we can pass whatever models we need in the array.
If we see the console, there will be a number 4 printed because we have four elements in our collection. As mentioned before, if we use the add
method, the new element will be placed in the last position of the collection, but what if we want to add the new element to the first position, or maybe somewhere else? We can use the insert
method to add the new element wherever we need.
Looping through the records/models in the store
So far, we know how to retrieve the number of elements in the existing store. Now we can iterate through the elements of the store by using the each
method as follows:
store.each(function(record, index){ console.log(index, record.get("name")); });
The each
method receives a function as the first parameter. This function will be executed for every record of the store; the anonymous function receives two parameters for our convenience: the record
and index
parameters for each iteration.
We can also set the scope where the anonymous function will be executed by passing a second parameter to the each
method with the object where the anonymous function will be executed.
In our previous example, we only printed the index
and name
properties in our model, but we can access any property or method defined in our Customer
model.
Retrieving the records in the store
Once we have content in our store, we can retrieve objects or perform a search of the collection of models. There are several ways of retrieving models. We are going to look at the most common ways.
By index position
If we only want to get a model at a specific position, we can use the getAt
method from the store as follows:
var modelTest = store.getAt(2);
console.log(modelTest.get("name"));
In our previous example, we get the model that is in the third position of the collection. The first position in the store uses the index 0
, so if we want to get the third element, we use the index 2
. In our example, the name printed should be Modern Cars of America.
First and last records
There are also methods to retrieve the first element of the collection and the last element; for this, we can execute the first
and last
methods from the store class, as shown in the following code:
var first = store.first(); var last = store.last(); console.log(first.get("name"), last.get("name"));
Our previous code will print the name of the first and last element in our store; in this case, we will see the name of Acme Corp and Extreme Sports Los Cabos.
By range
There are times when we need to get many records at once, so there's a method called getRange
to retrieve a list of records. We may define the limits, or we can even get all the records in the collection, as shown in the following code snippet:
var list = store.getRange(1,3);
Ext.each(list,function(record,index){
console.log(index,record.get("name"));
});
In the previous code, we were retrieving records from the index number 1
to the index number 3
. We are going to see three elements in our JavaScript console.
By ID
We can retrieve a record directly by its ID, as shown in the following code:
var record = store.getById(10001); console.log(modelTest.get("name"));
Removing records
We have been adding and accessing records in our store, but if we would like to remove records from the store, we'd have three ways of doing this task:
store.remove(record); store.each(function(record,index){ console.log(index,record.get("name")); });
We executed the remove
method and passed the model from where we wanted to delete the record. In our previous code, we were passing the model
variable that we created before. If we look at the JavaScript console, we will see that the first record does not exist anymore.
We can also remove many records at once. We only need to pass an array of models to the remove
method, and those models will be removed from the store, as shown in the following code:
store.remove([first,last]);
store.each(function(record,index){
console.log(record.get("name"));
});
When we execute the code, we will see that the two additional records are gone. We should not see those names in the JavaScript console.
There are times when we may not have the reference to the model that we want to delete. In those cases, we can remove a record by its position in the store, as shown in the following code:
store.removeAt(2);
store.each(function(record,index){
console.log(index,record.get("name"));
});
The removeAt
method accepts an index; the record located at this position will be removed. Now we can only see two names in the JavaScript console.
If we want to remove all the records in our store, we only need to call the removeAll
method and the store will be cleared.
store.removeAll();
console.log("Records:",store.count());
At this moment, our store is empty. If we execute the count
method, we will get zero as a result. Now we know how to add, retrieve, and remove records from our store.