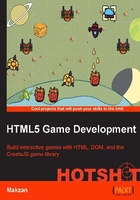
Creating the game scenes
In this task, we kick-start the project with game scenes such as the menu, game, and game-over condition, defined in a game-flow logic. We will also get these scenes linked together.
Prepare for lift off
We need to create a new directory for our new game project. Inside the project folder, we have the following:
- An
index.html
file for the view - A
game.css
file for the styling - A directory named
js
for all the logic files - A directory named
images
for all the game graphics - Lastly, we need the following four images placed in the
images
folder:
Engage thrusters
First of all, we start at where the web browser begins loading our game, that is, the index.html
file, and perform the steps given as follows:
- Put the following HTML code in the
index.html
file. Most of the tags and layout codes are similar to the ones we saw in Project 1, Building a CSS Quest Game:<!DOCTYPE html> <html lang='en'> <head> <meta charset='utf-8'> <title>Card Battle</title> <link rel="stylesheet" href="game.css"> </head> <body> <header> <div class="row"> <h1>Card Battle!</h1> </div> </header> <section id="game" class="row"> <!-- The game section starts --> <div id="start-scene" class="scene"> <a href="#" id="start-btn" class="button"></a> </div> <div id="game-scene" class="scene out"></div> <div id="gameover-scene" class="scene out won loss"> <p><a href="#" id="back-to-menu-button" class="button"></a></p> </div> </section> <!-- The game section ends --> <section class='how-to-play row'> <h2>How to Play?</h2> <p>Pick one card and watch the battle result. Beat opponent before you run out of HP.</p> </section> <footer> <div class="row"> <p>Copyright goes here.</p> </div> </footer> <!-- Loading all the JS logics --> <script src='js/patch.js'></script> <script src='js/scenes.js'></script> <script src='js/game-scene.js'></script> <script src='js/game.js'></script> </body> </html>
- The HTML only defines how the content and game elements are grouped and organized. The styling is always done in CSS. So, we have the following style rules defined in the
game.css
file:#game { width: 480px; height: 600px; border-radius: 8px; overflow: hidden; } .scene { width: 100%; height: 100%; position: absolute; overflow: hidden; border-radius: 8px; transition: all .3s ease-out; } .scene.out { transform: translate3d(100%, 0, 0); } .scene.in { transform: translate3d(0, 0, 0); } .button { position: absolute; width: 100%; height: 100%; top: 0; left: 0; } #start-scene { background: url(images/start-scene-bg.png);} #game-scene { background: url(images/battle-bg.png);} #gameover-scene.won { background: url(images/you-won.png);} #gameover-scene.loss { background: url(images/you-loss.png);}
- Now, we have the game elements and their styles defined. The next thing is the logic of toggling the different states of the game elements. This is the job of JavaScript. There are many different components in a game, and we will divide them into individual files for easier code readability. The following code controls the game flow and is placed in the
game.js
file:;(function(){ var game = this.cardBattleGame = this.cardBattleGame || {}; // Main Game Flow game.flow = { startOver: function() { game.startScene.show(); game.gameScene.hide(); game.gameOverScene.hide(); }, startGame: function() { game.startScene.hide(); game.gameScene.show(); game.gameOverScene.hide(); }, gameOver: function() { game.startScene.hide(); game.gameScene.hide(); game.gameOverScene.show(); } } // Entry Point var init = function() { game.startScene.setup(); game.gameScene.setup(); game.gameOverScene.setup(); } init(); })();
- Then, we move to define how each scene should behave. Scene management is a major component worth a dedicated file. We put the following code in the
scenes.js
file:;(function(){ var game = this.cardBattleGame = this.cardBattleGame || {}; // Generic Scene object. var scene = game.scene = { node: document.querySelector('.scene'), setup: function(){}, onShow: function(){}, // hook for child objects to use. show: function() { this.node.classList.remove('out'); this.node.classList.add('in'); this.onShow(); }, hide: function() { this.node.classList.remove('in'); this.node.classList.add('out'); } }; // Start Scene var startScene = game.startScene = Object.create(scene); startScene.node = document.getElementById('start-scene'); startScene.setup = function() { document.getElementById('start-btn').onclick = function(){ game.flow.startGame(); return false; }; }; // Gameover Scene var gameOverScene = game.gameOverScene = Object.create(scene); gameOverScene.node = document.getElementById('gameover-scene'); gameOverScene.setup = function() { document.getElementById('back-to-menu-button').onclick = function() { game.flow.startOver(); }; }; })();
- We just created the starting menu and the game-over scene. But where is the major game scene? This time the game scene will grow into a large block of code. In order to make it easier to maintain, we put the code into a new file named
game-scene.js
shown as follows:// game scene module ;(function(){ var game = this.cardBattleGame = this.cardBattleGame || {}; var gameScene = game.gameScene = Object.create(game.scene); gameScene.node = document.getElementById('game-scene'); gameScene.onShow = function() { setTimeout(game.flow.gameOver, 3000); } })();
That's all the code that we need to complete the objective of creating the game scenes.
Objective complete – mini debriefing
We have set up the HTML structure and game scenes as explained in the following sections.
HTML structure for scenes
The #game
element is the key element. Inside the game, we have scenes that contain game objects. We have three scenes in this game. The major scene is game-scene
which contains most of the card-battle objects. The following is the structure of the HTML nodes in our game:
#game #start-scene //Start button #game-scene //Game elements to be added later #gameover-scene //Startover button
HTML structure for game objects
In a Document Object Model (DOM)-based HTML game, we define game objects by using DOM elements.
Logic modules
There are several core modules in our game, such as game flow, scene, game scene, battle animation, and health point. In this task, we define the game flow module and the structure of the scenes module. In the future tasks, we are going to refine each module to make them work together.
Game flow
The game flow logic is heavily inspired from the CSS matching game in in this game, we simplified the flow to have only three states: start over, start game, and game over. When each flow is toggled, the corresponding scene is displayed and all other scenes are hidden.
Scenes' methods
Some of the commonly defined methods are the setup
and onShow
methods. The setup
method is used for a specific scene to initialize and the onShow
method is called every time the scene is shown by its show
method. It is provided as a hook for each scene to have logic every time the scene is displayed. For example, in the later stage, our game-over
scene will change between the win and loss screen during the onShow
method.