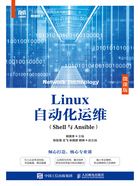
1.2.4 数据输入输出
在Shell脚本中,可以使用多种命令来实现数据输入输出功能,其中常用的命令有echo命令、printf命令、read命令。
1. echo命令

V1-4 数据输入输出
echo是一个常用的Shell命令。它的主要功能是输出字符串,可以将指定的文本字符串输出到标准输出(默认是屏幕),也可以用于输出提示信息、调试信息、结果信息等。echo命令的基本语法如下。
echo [options] string
其中,options表示可选的命令选项;string表示要输出的字符串。echo命令选项如表1-2所示。
表1-2 echo命令选项

使用以下命令输出字符串,当输出的字符串中包含空格或其他特殊字符时,通常使用引号标识字符串,例如:
[opencloud@server ~]$ echo "Hello, World!" Hello, World!
使用以下命令输出带有转义符的字符串,例如:
[opencloud@server ~]$ echo -e "Hello,\tWorld!" Hello, World!
在脚本中,可以使用echo命令输出变量的值,例如:
[opencloud@server ~]$ cat echo.sh #!/bin/bash name="John" age=18 echo "My name is $name,I am $age years old." # 执行脚本,输出结果如下 [opencloud@server ~]$ bash echo.sh My name is John,I am 18 years old.
在Shell中,echo命令支持一些常见的转义符,可以用来输出特殊字符。在使用转义符时,将字符串放在双引号之内。echo命令支持的转义符如表1-3所示。
表1-3 echo命令支持的转义符

使用echo命令和常见转义符的一些示例如下。
# 使用echo命令不换行输出字符串,继续在当前行输出 [opencloud@server ~]$ echo -e "Hello,\cWorld!" Hello, [opencloud@server ~]$ # 输出转义符 [opencloud@server ~]$ echo -e "\e" # 输出换页符 [opencloud@server ~]$ echo -e "Hello,\fWorld!" Hello, World! # 输出换行符 [opencloud@server ~]$ echo -e "Hello,\nWorld!" Hello, World! # 输出水平制表符 [opencloud@server ~]$ echo -e "Hello,\tWorld!" Hello, World! # 输出垂直制表符 [opencloud@server ~]$ echo -e "Hello,\vWorld!" Hello, World!
2. printf命令
在Shell脚本中,printf命令主要用于格式化输出字符串,输出带有特定格式的信息,如输出字符串、数字、字符、符号或者其他值。它与echo命令类似,但支持更多的格式化选项。
printf命令的基本语法如下。
printf format [argument...]
其中,format表示一个字符串,用于指定输出的格式,它可以包含转义序列,这些转义序列用于指定输出的格式和内容;argument表示一个或多个参数,用于提供要输出的内容。
printf命令需要在字符串中使用占位符,然后指定要输出的值。它可以通过在字符串中包含一些格式说明符,并按照格式说明符指定的格式将参数输出到标准输出。例如:
printf "%-10s %-8s %-4s\n" 姓名 性别 体重/kg printf "%-10s %-8s %-4.2f\n" 郭靖 男66.1234 printf "%-10s %-8s %-4.2f\n" 杨过 男68.6543 printf "%-10s %-8s %-4.2f\n" 郭芙 女47.9876
输出结果如下。
姓名 性别 体重/kg 郭靖 男 66.12 杨过 男 68.65 郭芙 女 47.99
在这个示例中,%-10s表示输出一个左对齐且宽度为10的字符串;%-8s表示输出一个左对齐且宽度为8的字符串;%-4.2f表示输出一个左对齐且宽度为4,小数点后保留2位的浮点数。
printf命令使用的占位符如表1-4所示。
表1-4 printf命令使用的占位符

续表

printf命令使用%c格式化字符的示例如下。
(1)输出单个字符,例如:
printf "The first letter of the alphabet is %c\n" 'a'
输出结果如下。
The first letter of the alphabet is a
(2)输出字符数组中的所有字符,例如:
characters=('a' 'b' 'c') printf "The characters are: %c %c %c\n" "${characters[@]}"
输出结果如下。
The characters are: a b c
(3)输出字符变量的值,例如:
letter='Z' printf "The letter is: %c\n" "$letter"
输出结果如下。
The letter is: Z
printf命令使用%s格式化字符串的示例如下。
(1)输出单个字符串,例如:
printf "The name of this website is %s\n" "Stack Overflow"
输出结果如下。
The name of this website is Stack Overflow
(2)输出字符串数组中的所有字符串,例如:
names=('Alice' 'Bob' 'Eve') printf "The names are: %s %s %s\n" "${names[@]}"
输出结果如下。
The names are: Alice Bob Eve
(3)输出字符串变量的值,例如:
greeting='Hello, world!' printf "The greeting is: %s\n" "$greeting"
输出结果如下。
The greeting is: Hello, world!
printf命令使用%d格式化整数的示例如下。
(1)输出单个整数,例如:
printf "The number is: %d\n" 42
输出结果如下。
The number is: 42
(2)输出整数数组中的所有数字,例如:
numbers=(1 2 3) printf "The numbers are: %d %d %d\n" "${numbers[@]}"
输出结果如下。
The numbers are: 1 2 3
(3)输出整数变量的值,例如:
count=5 printf "The count is: %d\n" "$count"
输出结果如下。
The count is: 5
printf命令使用%b格式化二进制整数的示例如下。
(1)输出单个二进制整数,例如:printf "The number is: %b\n" 5
输出结果如下。
The number is: 101
(2)输出二进制整数数组中的所有数字,例如:
numbers=(5 6 7) printf "The numbers are: %b %b %b\n" "${numbers[@]}"
输出结果如下。
The numbers are: 5 6 7
(3)输出二进制整数变量的值,例如:
binary=1101 printf "The binary number is: %b\n" "$binary"
输出结果如下。
The binary number is: 1101
printf命令使用%n输出字符总数的示例如下。
(1)在字符串中使用%n,例如:
printf "There are %d characters in this string.%n" 8 count echo "The value of count is: $count"
输出结果如下。
There are 14 characters in this string. The value of count is: 38
(2)在字符串数组中使用%n,例如:
strings=('This is string 1' 'This is string 2') printf "There are %d characters in string 1.%n" 100 count1 printf "There are %d characters in string 2.%n" 1000 count2 echo "The value of count1 is: $count1" echo "The value of count2 is: $count2"
输出结果如下。
There are 100 characters in string 1. There are 1000 characters in string 2. The value of count1 is: 37 The value of count2 is: 38
printf命令使用%(datefmt)T格式化日期和时间的示例如下。
(1)输出当前日期和时间,例如:
printf "The current date and time is: %(%Y-%m-%d %H:%M:%S)T\n"
输出结果如下。
The current date and time is: 2023-01-08 20:41:08
(2)输出使用自定义格式的日期和时间,例如:
printf "The date and time is: %(%a %b %d %I:%M %p %Z %Y)T\n"
输出结果如下。
The date and time is: Sun Jan 08 08:44 PM CST 2023
3. read命令
在Shell脚本中,read命令是一个内置命令,用于从标准输入(通常是键盘)中读取一行文本并将其赋值给一个或多个变量。该命令通常用于在脚本运行时从用户那里获取输入。
read命令的基本语法如下。
read [options] variable1 [variable2...]
其中,options表示可选的命令选项,可以用于指定输入的格式;variable1表示一个变量名,将保存读取的输入值;variable2表示可选变量,用于将输入的值赋给多个变量。
read命令常见命令选项如表1-5所示。
表1-5 read命令常见命令选项

使用read命令读取变量并输出。
[opencloud@server ~]$ cat read.sh #!/bin/bash read -p "What is your name? " name echo "Hello, $name"
执行脚本并查看输出结果。
[opencloud@server ~]$ bash read.sh What is your name? # 在命令行中输入tom jerry并按Enter键 Hello, tom jerry
在这个示例中,脚本会先输出一行提示,询问用户的名字。此后,脚本将等待用户输入名字,并将输入的值赋给变量name。最后,脚本将输出一行提示。
使用read命令向用户询问密码,并隐藏其输入的内容。
[opencloud@server ~]$ read -sp "Enter your password: " password Enter your password: #输入任何字符串,不会显示在屏幕上
在这个示例中,脚本会先输出一行提示,询问用户输入密码。此后,脚本将等待用户输入密码,输入的内容将被隐藏。最后,输入的值被赋给变量password。
使用read命令,向用户询问数字,只读取一个字符。
[opencloud@server ~]$ read -n 1 -p "Enter a number: " number Enter a number: 1
在这个示例中,脚本会先输出一行提示,询问用户输入数字。此后,脚本将等待用户输入一个字符,并将输入的值赋给变量number。