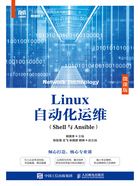
上QQ阅读APP看书,第一时间看更新
任务1.4.2 数据输入输出操作
1. 任务描述
编写Shell脚本,通过数据输入输出与用户交互,使用户输入数据或输出信息;通过数据输入输出读取文件中的数据或写入数据到文件中;通过数据输入输出可以与其他程序或系统交互,以获取或输出数据。使用read命令读取用户输入的数据,使用echo或printf命令输出信息。
2. 任务实施
(1)使用read命令读取用户输入的数据。
[opencloud@server ~]$ vim printf01.sh #!/bin/bash # 读入用户输入的数字 read -p "Enter a number: " num # 输出用户输入的数字 echo "You entered: $num" # 执行脚本并查看输出结果 [opencloud@server ~]$ bash printf01.sh Enter a number: 100 You entered: 100
(2)使用read命令读取多个数据。
[opencloud@server ~]$ vim printf02.sh #!/bin/bash # 读取多个数据 read -p "Enter your name, age and gender: " name age gender # 输出数据 echo "Name: $name" echo "Age: $age" echo "Gender: $gender" # 执行脚本并查看输出结果 [opencloud@server ~]$ bash printf02.sh Enter your name, age and gender: Tom 18 male Name: Tom Age: 18 Gender: male
(3)使用read命令读取文件中的每一行内容。
[opencloud@server ~]$ cat file.txt www.opencloud.fun www.redhat.com Linux Shell and Ansible [opencloud@server ~]$ vim printf03.sh #!/bin/bash # 读取文件中的每一行内容 while read line; do # 输出读取的每一行内容 echo $line done < file.txt # 执行脚本并查看输出结果 [opencloud@server ~]$ bash printf03.sh www.opencloud.fun www.redhat.com Linux Shell and Ansible
(4)使用printf命令格式化输出数字。
[opencloud@server ~]$ vim printf04.sh #!/bin/bash printf "%.2f\n" 3.14159265 printf "%.4f\n" 3.14159265 printf "%d\n" 123456 printf "%x\n" 255 # 执行脚本并查看输出结果 [opencloud@server ~]$ bash printf04.sh 3.14 3.1416 123456 ff
(5)使用printf命令格式化输出字符串。
[opencloud@server ~]$ vim printf05.sh #!/bin/bash printf "%-10s %-8s %-4s\n" Name Gender Age printf "%-10s %-8s %-4d\n" John Male 30 printf "%-10s %-8s %-4d\n" Mary Female 25 # 执行脚本并查看输出结果 [opencloud@server ~]$ bash printf05.sh Name Gender Age John Male 30 Mary Female 25
(6)使用printf命令输出多个字符和字符串。
[opencloud@server ~]$ vim printf06.sh #!/bin/bash printf "%s %s %s\n" A B C printf "%s %s %s\n" A B C D E F G # 定义字符变量 char='a' # 使用 %c格式化输出字符 printf "The character is %c.\n" $char # 定义字符串变量 string="Hello, World!" # 使用 %s格式化输出字符串 printf "The string is %s.\n" "$string" # 使用 %-10s格式化输出左对齐的字符串 printf "%-10s\n" "$string" # 执行脚本并查看输出结果 [opencloud@server ~]$ bash printf06.sh
(7)使用printf命令输出变量值。
[opencloud@server ~]$ vim printf07.sh #!/bin/bash name="John" age=30 printf "My name is %s, and I am %d years old.\n" "$name" "$age" # 上面的脚本中定义了两个变量:name和age # 使用printf命令输出字符串,并使用 %s和 %d占位符引用变量 # printf命令可以使用多个参数,使用变量时需使用$调用变量 # 执行脚本并查看输出结果 [opencloud@server ~]$ bash printf07.sh My name is John, and I am 30 years old.