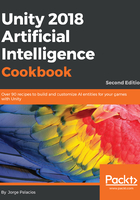
上QQ阅读APP看书,第一时间看更新
Getting ready
We need to make some modifications to our AgentBehaviour class:
- Add new member values to limit some of the existing ones:
public float maxSpeed; public float maxAccel; public float maxRotation; public float maxAngularAccel;
- Add a function called MapToRange. This function helps in finding the actual direction of rotation after two orientation values are subtracted:
public float MapToRange (float rotation) { rotation %= 360.0f; if (Mathf.Abs(rotation) > 180.0f) { if (rotation < 0.0f) rotation += 360.0f; else rotation -= 360.0f; } return rotation; }
- Also, we need to create a basic behavior called Align that is the stepping stone for the facing algorithm. It uses the same principle as Arrive, but only in terms of rotation:
using UnityEngine; using System.Collections; public class Align : AgentBehaviour { public float targetRadius; public float slowRadius; public float timeToTarget = 0.1f; public override Steering GetSteering() { Steering steering = new Steering(); float targetOrientation = target.GetComponent<Agent>().orientation; float rotation = targetOrientation - agent.orientation; rotation = MapToRange(rotation); float rotationSize = Mathf.Abs(rotation); if (rotationSize < targetRadius) return steering; float targetRotation; if (rotationSize > slowRadius) targetRotation = agent.maxRotation; else targetRotation = agent.maxRotation * rotationSize / slowRadius; targetRotation *= rotation / rotationSize; steering.angular = targetRotation - agent.rotation; steering.angular /= timeToTarget; float angularAccel = Mathf.Abs(steering.angular); if (angularAccel > agent.maxAngularAccel) { steering.angular /= angularAccel; steering.angular *= agent.maxAngularAccel; } return steering; } }